#3.0 Introduction
- 타입 문법과 함꼐하는 자바스크립트
- 추가 : 타입 체크, 자동 완성, 인터페이스, JSX 문법
- 언어 작동 전에 타입 확인함 => stongly-type
- 브라우저는 자바스크립트만 이해할 수 있음 => 타입스크립트는 이해 X
- 컴파일 시 자바스클비트로 변경 -> 실행 전에 타입 체크 하고 오류 알려줌
#3.1 DefinitelyTyped
CRA로 타입스크립트 프로젝트 생성
1. [npx or yarn] create-react-app [내 앱 이름] --template typescript
2. yarn add styled-components 로 스타일드 컴포넌트 먼저 설치
3. yarn add @types/styled-components -D 으로 타입스크립트 타입 정의 설치
** @types란? 큰 깃허브 레포. npm 패키지 저장소. 스타일컴포넌트의 타입.
사람들이 만들어 낸 타입 설명. Definitely Typed 저장소로부터 옴
타입스크립트에게 패키지가 무엇인지 설명해주기
#3.2 Typing the Props
- 타입스크립트에게 내 컴포넌트가 가져야 하는 prop 설명해주기
- 코드 실행 전에 타입 확인 원함
- 타입스크립트로 prop 보호하기
prop 객체 모양을 설명해주기 => interface
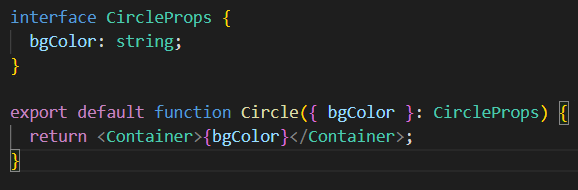
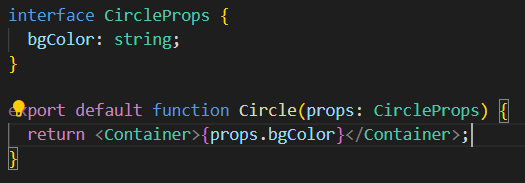
bgColor 는 CircleProps의 프로퍼티이다!
컴포넌트 프롭스 받아올 때 프롭스에 인터페이스 지정해주기
- 스타일 컴포넌트에게 props 타입 지정해주고 싶다면?
=> 똑같이 인터페이스 생성 후 div에 <인터페이스> 하여 타입 지정
즉 인터페이스는 객체가 구현해야 하는 프로퍼티와 메서드의 집합이자, 객체의 구조가 인터페이스에 정의된 구조와 일치하는지 확인하는 데 사용된다
#3.3 Optional Props
import Circle from "./Circle";
function App() {
return (
<div>
<Circle bgColor="teal" text="hello" />
<Circle bgColor="tomato" borderColor="teal" />
</div>
);
}
export default App;
import styled from "styled-components";
const Container = styled.div<CircleProps>`
width: 200px;
height: 200px;
border-radius: 100px;
display: flex;
justify-content: center;
align-items: center;
background: ${(props) => props.bgColor};
border: ${(props) => props.borderColor} 5px solid;
`;
interface CircleProps {
bgColor: string;
borderColor?: string;
text?: string;
}
export default function Circle({
bgColor,
borderColor,
text = "default text",
}: CircleProps) {
return (
<Container bgColor={bgColor} borderColor={borderColor ?? "tomato"}>
{text}
</Container>
);
}
- 속성을 옵셔널로 설정하고 싶다면, 속성명 뒤에 ?를 붙여준다
- 프롭을 넘겨줄 때 기본값을 지정해주고 싶을 경우 ?? 뒤에 기본값을 적어준다
- ES6 JS 문법 : 기본 매개변수 -> 함수를 호출할 때 해당 매개변수에 값을 전달하지 않을 때 사용할 기본값을 제공하는 구문
** ??는 JavaScript에서 사용되는 "Null 병합 연산자(Nullish Coalescing Operator)"입니다. 이 연산자는 좌측 피연산자가 null이나 undefined일 경우에만 우측 피연산자를 반환합니다. 만약 좌측 피연산자가 null 또는 undefined가 아니라면, 좌측 피연산자의 값을 그대로 반환합니다.
#3.4 State
useState < number > ( )
state의 type을 지정하려면 Generics안에 타입을 지정
일반적으로는 초기값을 지정하면 타입스크립트가 자동으로 타입을 유추하기 때문에 굳이 지정해주지 않아도 되지만 상태가 undefined또는 null이 될 수도 있거나 객체 또는 배열일 때는 지정해주는 것이 좋다.
ex) const [ value, setValue ] = useState< Value | null >(null);
(출처 : sugar님 댓글)
#3.5 Forms
import { useState } from "react";
function App() {
const [value, setValue] = useState("");
const onChange = (event: React.FormEvent<HTMLInputElement>) => {
const value = event.currentTarget.value;
// > const {value} = event.currentTarget
// > const {currentTarget:{value}} = event
setValue(value);
};
const onSubmit = (event: React.FormEvent<HTMLFormElement>) => {
event.preventDefault();
console.log("hello, ", value);
};
return (
<div>
<form onSubmit={onSubmit}>
<input
value={value}
onChange={onChange}
type="text"
placeholder="username"
/>
<button>Login</button>
</form>
</div>
);
}
export default App;
#3.6 Themes
Styled-components (TypeScript)
DefaultTheme는 기본적으로 props.theme의 인터페이스로 사용됩니다.
기본적으로 DefaultTheme 인터페이스는 비어 있으므로 확장해야 합니다.
// import original module declarations
import "styled-components";
// and extend them!
declare module "styled-components" {
export interface DefaultTheme {
textColor: string;
bgColor: string;
btnColor: string;
}
}
https://styled-components.com/docs/api#typescript
(출처 : sugar님 댓글)
#3.7 Recap
SyntheticEvent (합성 이벤트)
이벤트 핸들러는 모든 브라우저에서 이벤트를 동일하게 처리하기 위한 이벤트 래퍼 SyntheticEvent 객체를 전달받습니다.
Keyboard Events
ex) onKeyDown onKeyPress onKeyUp
Focus Events
ex) onFocus onBlur
Form Events
ex) onChange onInput onInvalid onReset onSubmit
Generic Events
ex) onError onLoad
etc..
https://reactjs.org/docs/events.html
(출처 : sugar님 댓글)
'강의 > 노마드코더' 카테고리의 다른 글
[React JS 마스터클래스] #2 STYLED COMPONENTS 정리 (0) | 2024.01.21 |
---|